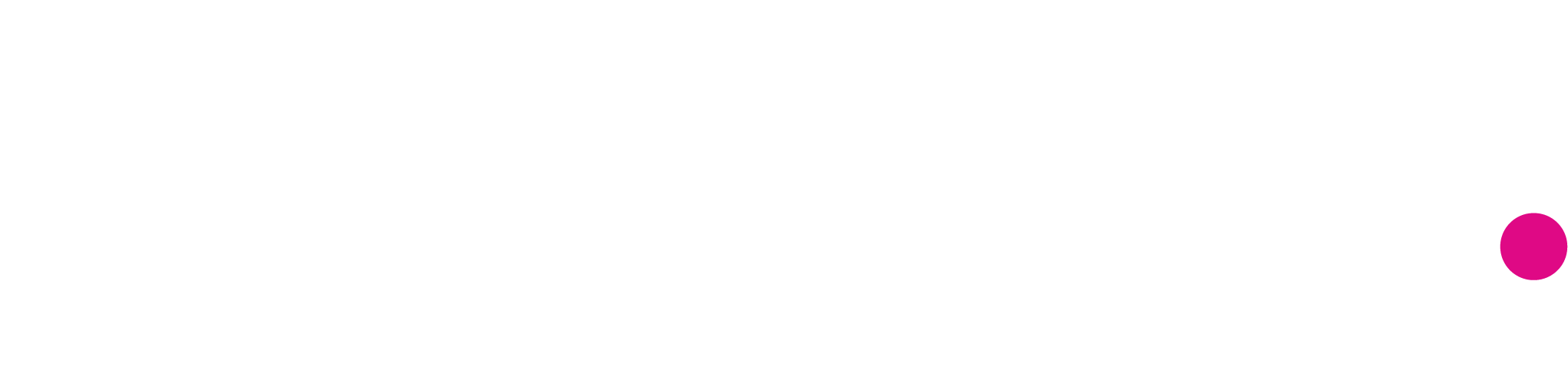
Getting Started
Authentication
The /auth/authenticate
endpoint allows you to obtain an authorization bearer token required for making authenticated requests to the API. This guide provides step-by-step instructions on how to use this endpoint.
Prerequisites
Before proceeding, ensure that you have:
Subscription Key:
Can be used as a Query Parameter: subscription-key or Header: Ocp-Apim-Subscription-Key
The subscription key will be specific to the environment you are connecting to, ie sandbox, sandbox1, sandbox2, production.
API Credentials:
apiKey
: Your API key.apiSecret
: Your API secret.
Steps to Obtain Authorization Token
1. Make a POST Request to /auth/authenticate
Send a POST request to the /auth/authenticate
endpoint with the following details:
Endpoint:
/auth/authenticate
Method: POST
Request Headers: Ocp-Apim-Subscription-Key: your_subscription_key
(Ensure the Content-Type is set to application/json)
Request Body:
{ "apiKey": "your_api_key", "apiSecret": "your_api_secret" }
2. Receive Authorization Token
If the request is successful, you will receive a response with a status code of 200
. The response body will contain an authorization token and its expiration date.
{ "token": "your_authorization_token", "expiration": "expiration_date_time" }
Important Notes
Security: Keep your API key and secret secure. Do not expose them in public spaces.
Token Expiration: The authorization token has a limited lifespan. Be aware of its expiration and refresh it as needed.
3. Use the Authorization Token
Include the obtained authorization token in the Authorization header of your subsequent API requests. This will authenticate and authorize your requests to the protected endpoints.
Test by making a GET Request to /account/list
Send a GET request to the /account/list endpoint with the following details:
Endpoint: /account/list
Method: GET
Request Headers:
Authorization: Bearer your_authorization_token
Ocp-Apim-Subscription-Key: your_subscription_key
If the request is successful, you will receive a response with a status code of 200. The response body will contain a JSON object with information about the listed accounts.
{ "success": true, "error": "string", "message": "string", "count": 0, "data": [ { "accountId": "string", "accountNumber": "string", "productName": "string" }, // Additional account entries may be present ] }
Idempotency
Idempotency is a concept in API design that ensures the same request, when made multiple times, produces the same result as the initial request. It is particularly useful in scenarios where a client may need to retry a request that was not completed successfully without risking unintended side effects.
This guide explains the use of the Idempotency-Key
header in API requests to achieve idempotency.
What is the Idempotency-Key?
The Idempotency-Key
is a unique value generated by the client and included in the request headers. This key allows the server to recognize subsequent retries of the same request and ensures that repeated requests with the same key do not result in unintended side effects.
How to Use Idempotency-Key
1. Generate a Unique Idempotency Key
Before making an API request, generate a unique idempotency key. This key should be unique for each distinct operation or request.
2. Include the Idempotency Key in the Request
Include the generated idempotency key in the Idempotency-Key
header of your API request. This header signals to the server that the request should be treated idempotently.
POST /api/endpoint Host: api.bankprov.com/
payment/achContent-Type: application/json Idempotency-Key: unique_idempotency_key
3. Server Processing
When the server receives a request with the Idempotency-Key
header, it checks if a previous request with the same key has been processed in the past 12 hours. If the server has already processed a request with the same key, it returns an error:
You have provided a duplicate Idempotency key. (Error Code: VE00027)
ACH
Lots or resources are available in BankProv's ACH Originator Guide
Reversals
Introduction to ACH Reversals
Definition: An ACH reversal is used to correct an erroneous or duplicate transaction that has been processed through the ACH network. It effectively cancels the original transaction, either fully or partially, depending on the specific situation.
Purpose: The primary purpose of an ACH reversal is to rectify processing errors, ensuring the financial integrity of both parties involved in the transaction.
When to Use an ACH Reversal
Duplicate Transactions: If a transaction is accidentally processed more than once, an ACH reversal can be used to remove the duplicate entries. While we utilize idempotency to prevent duplicate transactions if a duplicate is submitted with unique idempotency keys a reversal can be used.
Incorrect Amounts: In cases where the transaction amount is wrong, a reversal can correct the financial records.
Wrong Account: If funds are sent to or debited from the wrong account, a reversal file can rectify this error.
Unauthorized Transactions: For transactions processed without proper authorization, reversals are necessary to comply with legal and regulatory standards.
Legal and Compliance Considerations
Timeframe: The National Automated Clearing House Association (NACHA) rules generally require reversals to be initiated within five business days from the settlement date of the erroneous transaction.
Authorization: Certain reversals might require the consent of the party receiving the funds, depending on the nature of the error and regulatory requirements.
To Submit an ACH Reversal through the API
Submit a new ACH Payment throught the correct /payment endpoint
Ensure the values for counterparty and other details match the original transaction.
Use: "REVERSAL__" as the companyEntryDescription property.
***Failure of an Originator or Third-Party Sender to fund its outgoing credit file is not a valid reason for a file reversal.***
The reversing must be transmitted to the bank within five banking days after the settlement date of the duplicate/erroneous file.
Transmit the reversing within 24 hours of discovering the error.
OUR TEAM MAY REACH OUT TO YOU TO ASSIST IF A REVERSAL IS SUBMITTED
Webhooks
Data for the chosen events will be sent as JSON via POST requests to the provided URLs.
Format
{ "PaymentId": "a85ab18d-cb35-468f-bb0e-d5e07dbeb675", "OldStatus": "Pending", "NewStatus": "Approved", "EffectiveDate": "2024-01-11" }
X-ProvX-Signature Value
49a354dac40cb896f4a49afe1201239d1e83952a
Verifying Webhooks from Our System
Understanding the Verification Process
To ensure the security and integrity of webhooks received by your system, it's crucial to verify the source of each webhook request. This is done using the "X-ProvX-Signature" header, which is included in each webhook request sent from our system.
X-ProvX-Signature Header
Purpose: The "X-ProvX-Signature" header is used to validate the authenticity of the webhook request.
Composition: This header contains a SHA1 hash. This hash is a unique representation, generated from the concatenation of the webhook ID and the JSON body of the request.
Steps for Verification
Extract the Signature:
When your system receives a webhook request, extract the "X-ProvX-Signature" header value.
Generate Your Hash:
Use the SHA1 hashing algorithm to create a hash. Concatenate the webhook ID and the JSON body of the request (in that order) to form the input string for the hash.
Compare Hashes:
Compare the SHA1 hash you generated with the hash provided in the "X-ProvX-Signature" header. If they match, it confirms the request is from a trusted source.
Webhook Events
Below are the events available for triggering a webhook.
Payment Received
Triggered when a payment is successfully received by your system.
Payment Status Update
Notifies changes in the status of a payment.
Sub-Events:
ACH Statuses:
Completed: Indicates the payment has been processed and sent to the Federal Reserve.
Settled: Occurs when funds are deposited into the account.
Returned: Triggered when funds are returned by the other institution.
Wire Completed
Indicates the completion of a wire transfer.
ProvX Completed
Triggered upon the successful completion of a ProvX transaction.
Account Application Status Update
Notifies changes in the status of an account application.
Account Created
Triggered when a new account is successfully created.
Account Fee
Notifies when a fee is applied to an account.
Customer Person Verification Status Update
Updates on the verification status of an individual customer.
Customer Business Verification Status Update
Updates on the verification status of a business customer.